In the ever-evolving landscape of web development, managing state in a complex application can be a daunting task. This is where Redux steps in as a powerful state management library that simplifies the process of handling data across your application. Whether you're a seasoned developer or just starting your journey into web development, understanding Redux is crucial for building scalable and maintainable applications. In this comprehensive guide, we'll delve deep into what is Redux, how it works, its core principles, and why it's so essential in modern web development. The State of State ManagementBefore we dive into Redux, let's take a moment to explore the need for state management in web applications. 1.1 State in Web DevelopmentWeb applications are inherently dynamic. They constantly respond to user interactions, server responses, and other real-time events. To maintain a consistent user experience, developers need a way to manage and update the application's data or state efficiently. 1.2 Challenges with State ManagementAs web applications grow in complexity, managing state becomes increasingly challenging. Here are some common issues developers face: 1.2.1 Prop DrillingPassing state down through multiple components can lead to "prop drilling," where intermediate components need to pass data to their child components even if they don't use it directly. 1.2.2 Global StateIn some cases, you need to share state across different parts of your application. Global state management becomes essential but is often cumbersome to implement. 1.2.3 Predictable State ChangesMaintaining the predictability of state changes is crucial to prevent bugs and ensure a smooth user experience. 1.3 Enter ReduxRedux was created to address these challenges. It's a state management library for JavaScript applications, primarily used with frameworks like React. Redux provides a predictable and centralized way to manage the state of your application. Understanding Redux FundamentalsTo grasp Redux fully, you need to understand its core concepts and how they work together. Let's start with the building blocks of Redux. 2.1 ActionsActions are payloads of information that describe an event in your application. They are the only source of data in a Redux application. 2.1.1 Action TypesEach action type is defined as a string constant, describing the type of action being performed. For example, you might have an action type like "ADD_TODO" to represent adding a new todo item. 2.2 ReducersReducers are pure functions that specify how the application's state changes in response to actions. They take the current state and an action as arguments and return a new state. 2.2.1 ImmutabilityReducers must always return a new state object rather than modifying the existing state. This ensures predictability and simplifies debugging. 2.3 StoreThe store is the central repository for your application's state. It holds the current state, provides a way to dispatch actions, and allows you to subscribe to state changes. 2.3.1 Single Source of TruthWith Redux, you have a single source of truth for your application's state, making it easier to debug and reason about your code. 2.4 MiddlewareMiddleware in Redux is a way to extend its functionality. It sits between dispatching an action and the moment it reaches the reducer. Middleware can be used for various purposes, such as logging, async operations, and routing. 2.5 React and Redux IntegrationRedux is commonly used with React, thanks to libraries like "react-redux" that simplify the integration process. This combination allows for a seamless flow of data between React components and the Redux store. The Redux WorkflowTo illustrate how Redux works in practice, let's walk through a typical workflow using Redux. 3.1 Action CreationWhen an event occurs in your application, such as a user clicking a button, you create an action. This action describes what happened and may include additional data. 3.2 Dispatching ActionsTo make changes to the state, you dispatch the action using the Redux store's method. This action is then sent to all registered reducers.dispatch 3.3 Reducer HandlingReducers specify how the state should change in response to the action. They are pure functions, which means they don't have side effects and always return a new state. 3.4 State UpdateThe reducer returns the new state, which is stored in the Redux store. All components subscribed to the relevant part of the state will receive updates. 3.5 React Component InteractionIn a React component, you can connect to the Redux store using the function provided by "react-redux." This allows your component to access the state and dispatch actions.connect Benefits of Using ReduxNow that you have a good understanding of Redux's core concepts and workflow, let's explore the benefits of using Redux in your applications. 4.1 Predictability and DebuggingRedux enforces a strict unidirectional data flow, making it easier to trace the source of bugs and understand how your application's state changes over time. 4.2 ScalabilityAs your application grows, Redux provides a scalable architecture for managing state. It remains effective even in large, complex applications. 4.3 ReusabilityRedux promotes the separation of concerns and the creation of reusable components. Actions and reducers can be shared and reused across different parts of your application. 4.4 DevTools IntegrationRedux offers powerful development tools, such as the Redux DevTools Extension, which provides insights into your application's state changes and allows you to time-travel through state history. When to Use ReduxWhile Redux offers many benefits, it's essential to understand when it's appropriate to use. Not every project requires Redux, and using it unnecessarily can add complexity to your codebase. 5.1 Small and Simple AppsFor small, simple applications with minimal state management needs, Redux might be overkill. Consider using React's built-in state management instead. 5.2 Local Component StateIf the state you're managing is isolated to a single component and doesn't need to be shared with other parts of the application, using local component state is often sufficient. 5.3 Alternative State Management LibrariesThere are alternative state management libraries like Mobx and Recoil, each with its strengths and weaknesses. Evaluate your project's specific requirements before choosing a state management solution. Best Practices and TipsTo make the most out of Redux, it's crucial to follow best practices and consider some tips for a smoother development experience. 6.1 Organize Your CodeOrganize your Redux codebase into separate folders for actions, reducers, and components. This makes it easier to locate and maintain specific parts of your application. 6.2 Keep Reducers PureEnsure that your reducers are pure functions. Avoid side effects like API calls or asynchronous operations within reducers. 6.3 Use SelectorsSelectors are functions that extract specific pieces of data from the Redux store. They help encapsulate access to the store and improve performance by preventing unnecessary re-renders. 6.4 Middleware WiselyWhen using middleware, choose the ones that best suit your needs. Common middleware includes Redux Thunk for async actions and Redux Logger for debugging. Advanced Redux ConceptsOnce you've mastered the basics, you can explore advanced Redux concepts that enhance your state management capabilities. 7.1 Redux ToolkitRedux Toolkit is an official package recommended by the Redux team. It simplifies many aspects of Redux, including creating reducers, actions, and the store configuration. 7.2 Redux-SagaRedux-Saga is a middleware library that helps manage side effects in Redux applications. It's particularly useful for handling complex asynchronous logic. 7.3 ReselectReselect is a library for creating memoized selectors. It optimizes the performance of your application by preventing unnecessary computations. Real-World ApplicationsTo solidify your understanding of Redux, let's explore how it's used in real-world applications. We'll look at a few examples from various domains, such as e-commerce, social media, and productivity apps. 8.1 E-Commerce StoreLearn how Redux can manage the state of a shopping cart, product listings, and user authentication in an e-commerce application. 8.2 Social Media FeedDiscover how Redux can handle real-time updates and user interactions in a social media feed, including likes, comments, and posts. 8.3 Productivity AppExplore how Redux can be used to manage tasks, schedules, and notifications in a productivity app. ConclusionIn this comprehensive guide, we've covered everything you need to know about Redux, from its core concepts and workflow to its benefits, best practices, and advanced topics. Redux provides a robust and scalable solution for managing state in your web applications, making it an essential tool for modern web development. By mastering Redux, you'll be well-equipped to build maintainable, efficient, and reliable web applications that can handle the challenges of today's dynamic digital landscape. Start integrating Redux into your projects and unlock the full potential of state management in web development. And finally, if you're looking for expert React development services, consider reaching out to CronJ, a leading hire reactjs development company.
Create Date : 07 กันยายน 2566 |
Last Update : 7 กันยายน 2566 17:32:08 น. |
|
0 comments
|
Counter : 348 Pageviews. |
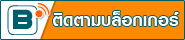 |
|